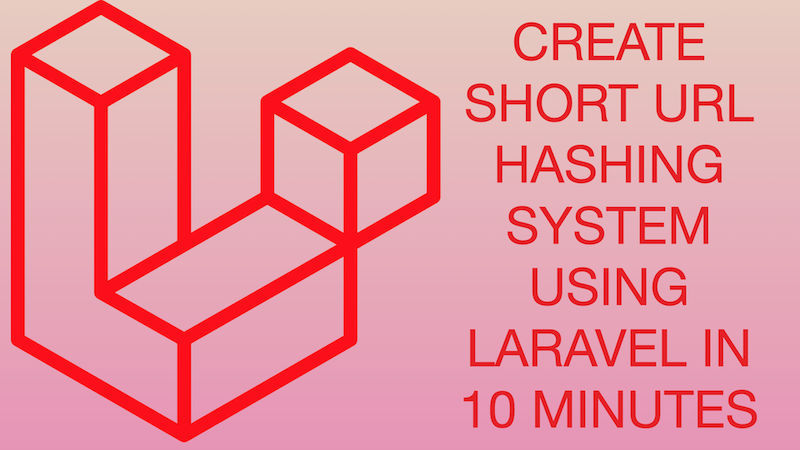
Last Updated On - March 3rd, 2024 Published On - Feb 08, 2023
Overview
In this Laravel short URL generator project we will learn, What is URL hashing? How it is used to create unique identifiers for web pages? We will also learn how we can track the number of clicks hit on a particular hashed URL.
What is URL hashing?
Hashing is a technique that involves taking a string of characters and using a cryptographic hash algorithm to create a unique numerical representation for the string. This numerical representation can then be used as a unique identifier for any webpage(particular reason) and can be used to access the webpage.
Architecture
I am implementing this URL hashing system as a REST API using the PHP Laravel framework. Laravel provides a great deal of built-in functionality for handling HTTP requests, routing, and database interactions, which will make it easier to build this application.
The core of the URL hashing system will be a hash function that takes an input URL and generates a unique, shortened hash. This hash will be stored in a database along with the original URL. When a request is made for the hashed URL, the application will look up the original URL in the database and redirect the user to it.
In order to track clicks, I will store the number of clicks for each hashed URL in the database and increment it every time the URL is accessed. I will also store the date the URL was created and when it was last clicked(accessed).
Steps To Implement
- Create a Laravel project using the following command:
composer create-project --prefer-dist laravel/laravel url-hashing-system
- Create a URL model with migration using the following command:
php artisan make:model Url -m
- In the
Url
model migration, add the following fields:original_url
: text field to store the original URLhash
: string field to store the hashed URLclicks
: integer field to store the number of clicks on the URL
- Create a controller to handle the URL hashing logic using the following command:
php artisan make:controller UrlController
- In the
UrlController
class, create a method to generate a hash for the URL and save it to the database:
public function generateHash(Request $request)
{
try {
$originalUrl = $request->input('url');
// Validating the URL
$request->validate([
'url' => 'required|url',
]);
// Creating Hash value of URL and stripping it upto 6 characters only
$hash = substr(hash('sha256', $originalUrl),0,6);
$url = Url::firstOrNew(
['original_url' => $originalUrl],
['hash' => $hash]
);
// Save the URL with its Hash Value
$url->save();
if ($url->id) {
return response()->json([
'hash' => url($hash)
], 201);
} else {
return response()->json([
'message' => 'Something goes wrong. Please try after sometime.'
], 500);
}
} catch (ValidationException $e) {
// Throwing Error in case of URL is empty or not valid
return response()->json([
'error' => $e->getMessage(),
], 400);
}
}
- Create a method in the controller to redirect the user to the original URL when the hashed URL is clicked:
public function redirect($hash)
{
// Get the Original URL on the basis of Hash.
$url = Url::where('hash', $hash)->first();
if (!$url) {
return response()->json([
'error' => 'URL not found'
], 404);
}
// Increase click counter By 1
$url->update([
'clicks' => $url->clicks + 1
]);
// Redirect to original URL
return redirect($url->original_url);
}
- Add the following routes in
routes/web.php
:
// Generating Hash
Route::post('/generate-hash', 'UrlController@generateHash');
// Redirection
Route::get('/{hash}', 'UrlController@redirect');
- Run the migration using the following command:
php artisan migrate
This implementation will allow us to generate a hash for any URL and store it in the database. The hash can be used as a shortened URL that can be shared and clicked, which will redirect the user to the original URL and track the number of clicks. This implementation can also be extended to make the URLs single use only by adding a flag in the database to track if a URL has already been used.
Also Read: Setting Up and Configuring Supervisor for Process Management on AWS EC2 Ubuntu
Setups To Clone the Up and Running Project
- Clone the repository to your local machine
git clone https://github.com/prashant12it/url-hashing-system.git
- Change into the newly created directory
cd url-hashing-system
- Install dependencies
composer install
- Copy the example environment file and configure your database settings
cp .env.example .env
- Generate an application key
php artisan key:generate
- Run database migrations
php artisan migrate
- Start the development server
php artisan serve
Also Read: Learn How to Use the Slack API to Post Messages in Slack Channel Using Laravel
Assumptions
- The input URL does not contain any malicious characters that would break the hash function.
- The target server is running PHP 7.4 or higher.
API Endpoints
The following endpoints will be available for the URL hashing system:
1. Hash a URL
POST /generate-hash
Request Body
{
"url": "https://www.newsatic.com/news/proposed-changes-in-new-tax-regime/"
}
Response
{ "hash": "http://127.0.0.1:8000/809efd" }
2. Redirect to Original URL
GET /{hashed_url}
Response
Redirects to the original URL associated with the given hashed_url.
3. Get Click Data
GET /get-click-report/{hash}
Response
{
"original_url": "https://www.newsatic.com/news/proposed-changes-in-new-tax-regime/",
"hash_url": "http://127.0.0.1:8000/809efd",
"total_clicks": 2,
"last_clicked": "2023-02-03 11:27:32"
}
Also Read: Sending messages to Microsoft Teams Channel by using Laravel
Deployment
Assuming you have a server with PHP and a web server (such as Apache or Nginx) installed, you can deploy the URL hashing system using the following steps:
- Copy the contents of the url-hashing-system directory to your server.
- Configure your web server to serve the contents of the public directory as the document root.
- Set the necessary environment variables, such as the database connection settings, on the server.
- Run the necessary database migrations.
Also Read: Laravel Image Resize Project in 10 Minutes: A Step By Step Guide
FAQs
Is there a difference between URL shortening and Hashed URLs?
Absolutely! While both shorten URLs, Hashed URLs offer additional benefits. Traditional URL shorteners generate random strings, making them unreadable and uninformative. Hashed URLs, on the other hand, create unique codes based on the original URL, maintaining some level of meaning and boosting click-through rates (CTR).
What are the benefits of using Hashed URLs over traditional Long URLs?
Long URLs can be clunky and unprofessional. Hashed URLs offer several advantages:
👉 Increased Aesthetics: Short, clean Hashed URLs enhance the visual appeal of your project.
👉 Improved Click Tracking: Track clicks more effectively with Hashed URLs compared to generic shortened links.
👉 Potential SEO Benefits: Concise URLs might be viewed more favorably by search engines (ongoing research).
👉 Enhanced Brand Recall: Shorter, memorable Hashed URLs can improve brand recognition.
Can I track clicks on Hashed URLs?
Yes! With the implementation explained in this guide, you can track clicks on your Hashed URLs. The system stores the original URL and tracks redirects, providing valuable click data.
How secure are Hashed URLs?
Hashed URLs themselves don’t inherently store sensitive information. While the hash reveals some information about the original URL length, it doesn’t expose the actual content. However, for maximum security, ensure the underlying system storing the original URL is secure.
Does this method work with other frameworks besides Laravel?
The core concept of Hashed URLs can be adapted to other frameworks. However, the specific implementation steps might vary depending on the framework’s functionalities.
What if I want to customize the Hashed URL format?
There might be limited customization options within Laravel’s base functionalities. However, exploring advanced Laravel features or third-party packages could provide more control over the Hashed URL format.
Are there any limitations to using Hashed URLs?
While beneficial, Hashed URLs have some limitations:
👉 Reduced Readability: Compared to the original URL, Hashed URLs might be less informative at first glance.
👉 Potential Confusion: Users unfamiliar with Hashed URLs might be hesitant to click.
How can I implement Hashed URLs in my project?
This guide provides a comprehensive step-by-step process for implementing Hashed URLs using Laravel. Follow the instructions and code examples to integrate this functionality into your project.
Also Read: Javascript Interview Questions For Frontend Developers 2024 – Part 1
TODO Next
- Store hashed URLs and their metadata in a separate database table with appropriate access controls to make the generated URLs privacy-aware
- Add tests for URL hashing functionality
- Add authentication for accessing click tracking data
- Implement expiration for hashed URLs
- Implement UI for generating hashed URLs
- Validate input URL for malicious characters that would break the hash function
Also Read: Javascript Interview Questions 2024 – Part 2
Conclusion
This post has explored the concept of Hashed URLs and provided a step-by-step guide on implementing them using Laravel. Hashed URLs offer several advantages over traditional long URLs, including improved aesthetics, better click tracking and potential security benefits. If you’re looking for a way to enhance your Laravel project’s functionality and user experience, then implementing Hashed URLs is a great option to consider.
Want to see more of my projects?
Check out my portfolio for a wider range of my web development work: Prashant Web Developer