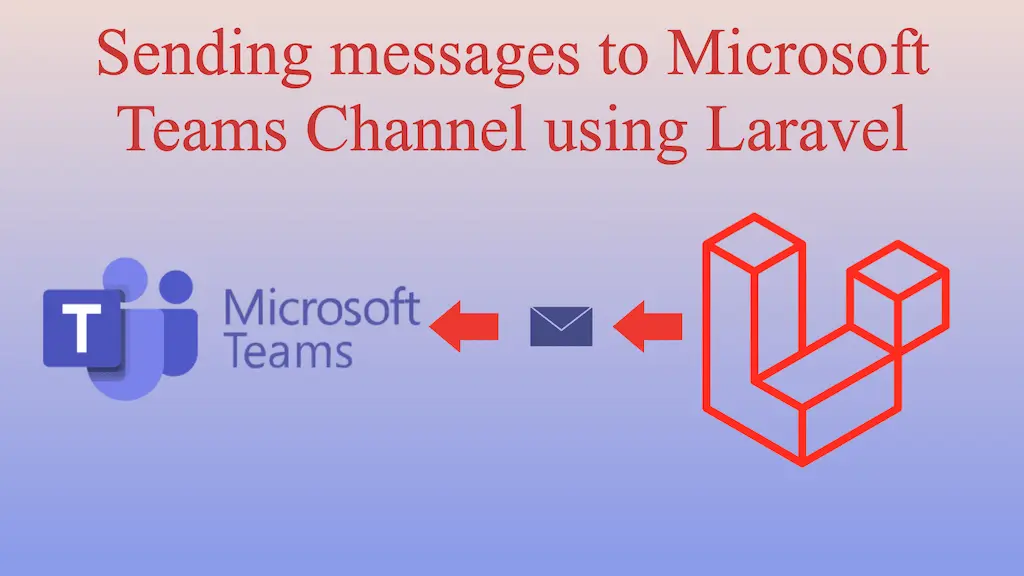
Last Updated On - August 2nd, 2024 Published On - Jun 05, 2023
To send a notification to Microsoft Teams channel using Laravel 10, you can use laravel-notification-channels/microsoft-teams package. In this post, I’ve explained step by step process for integrating and implementing this php package.
Expected Result
Create Teams channel and webhook URL
1. Open Microsoft Teams, go to the Teams menu and create a new team.
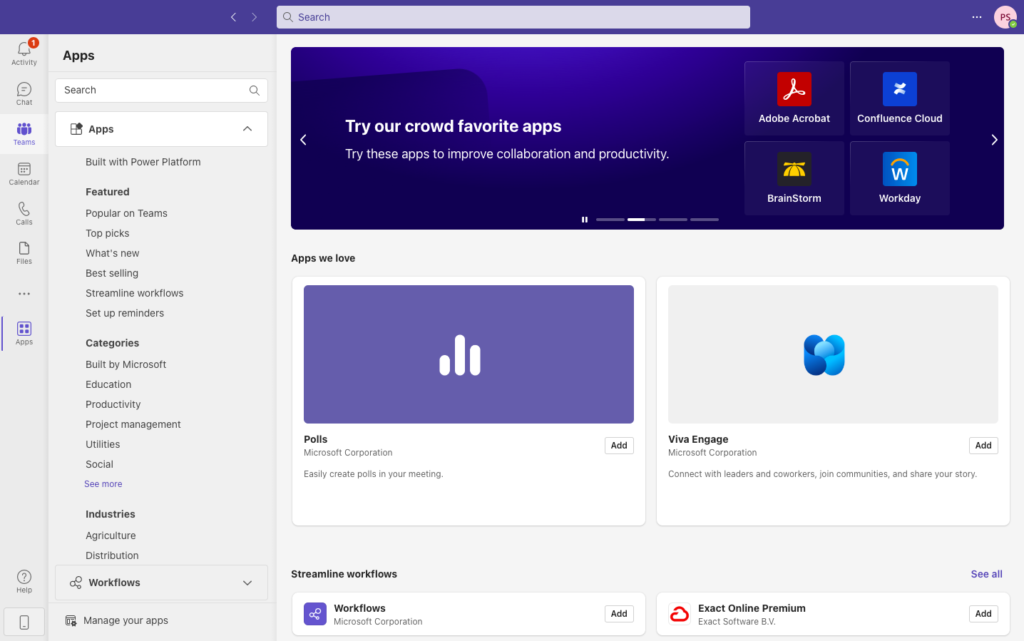
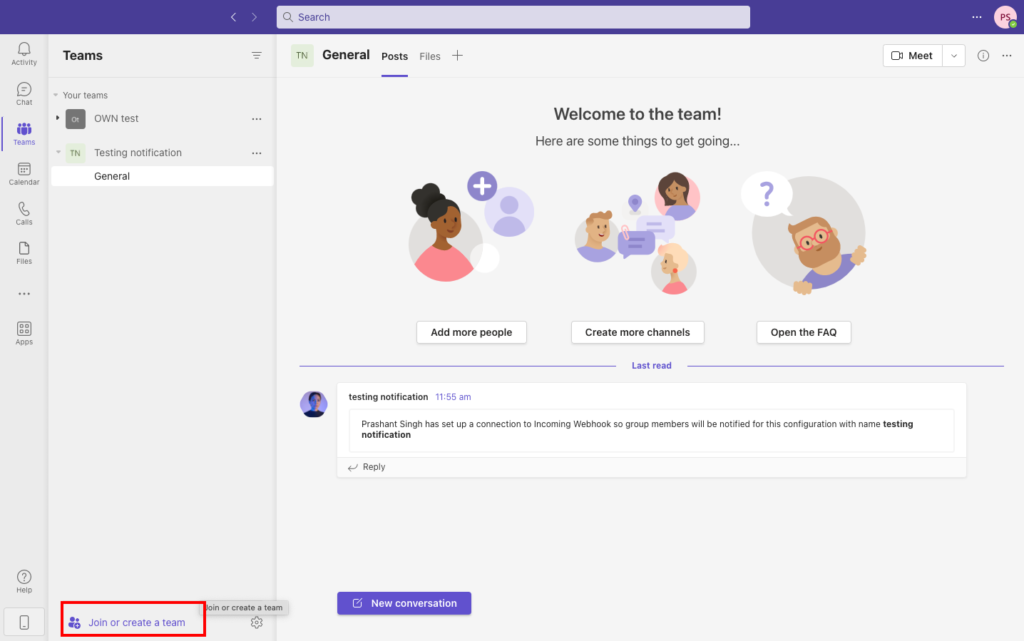
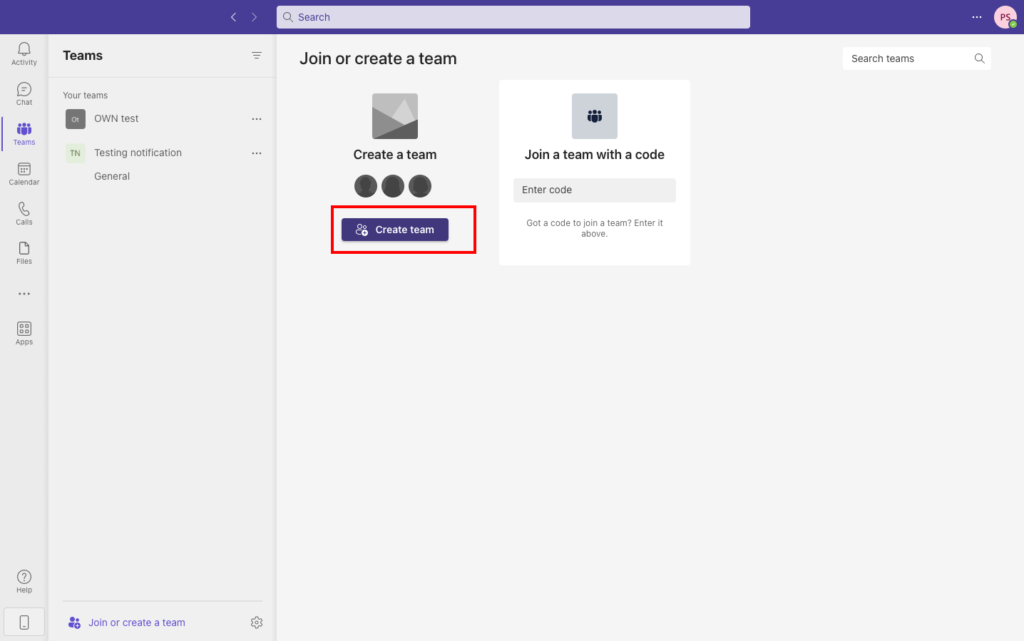
2. Select From scratch option to create the channel.
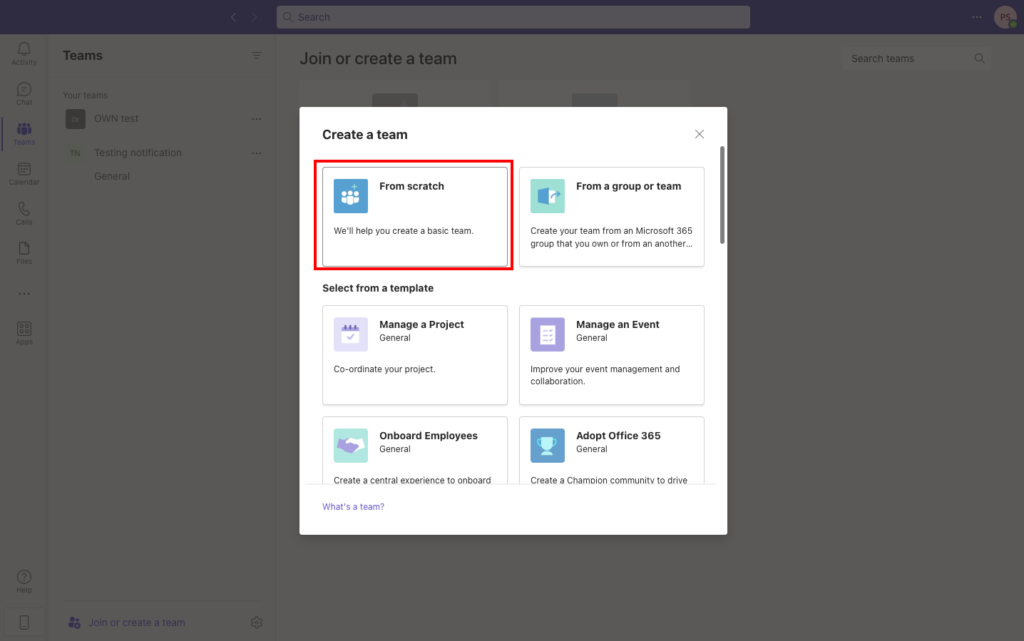
3. Choose whether your channel is publicly accessible or it is a private channel. Enter the title and description of the channel and click next. Your channel is now created.
4. You can see your channel on the left-hand sidebar in Teams. Click on the 3 dots of the channel general, you’ll see couple of options there. You have to select Manage channel. On the main pannel click on the 3 dots at the top, this will again show a couple of options but you’ve to choose connectors this time.
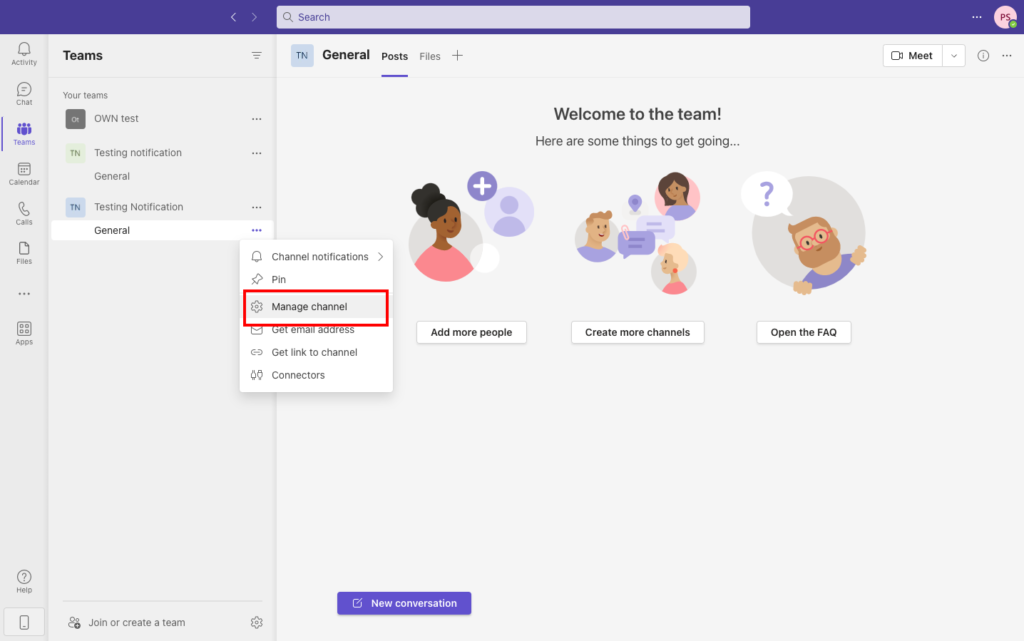
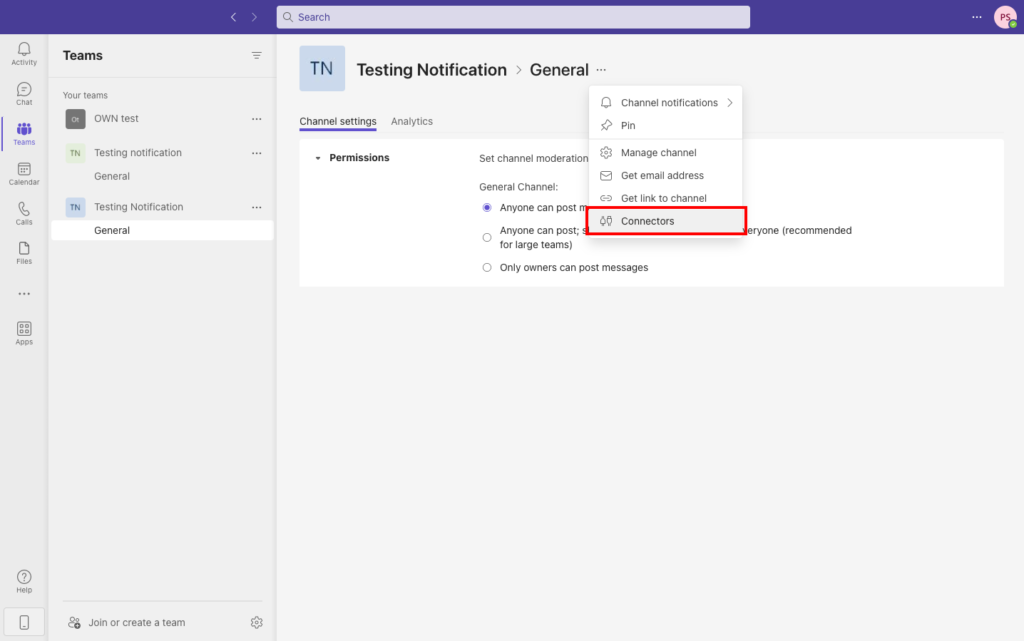
5. Search Incoming Webhook connector and add it to your channel. Go to the general 3 dot menu and again click on connectors. This time you have to select configure option which is visible in front of Incoming Webhook. Give a name to your webhook. Upload the logo if you have any for the channel and click on create button. This will give you a webhook URL which we’ll add to the laravel code.
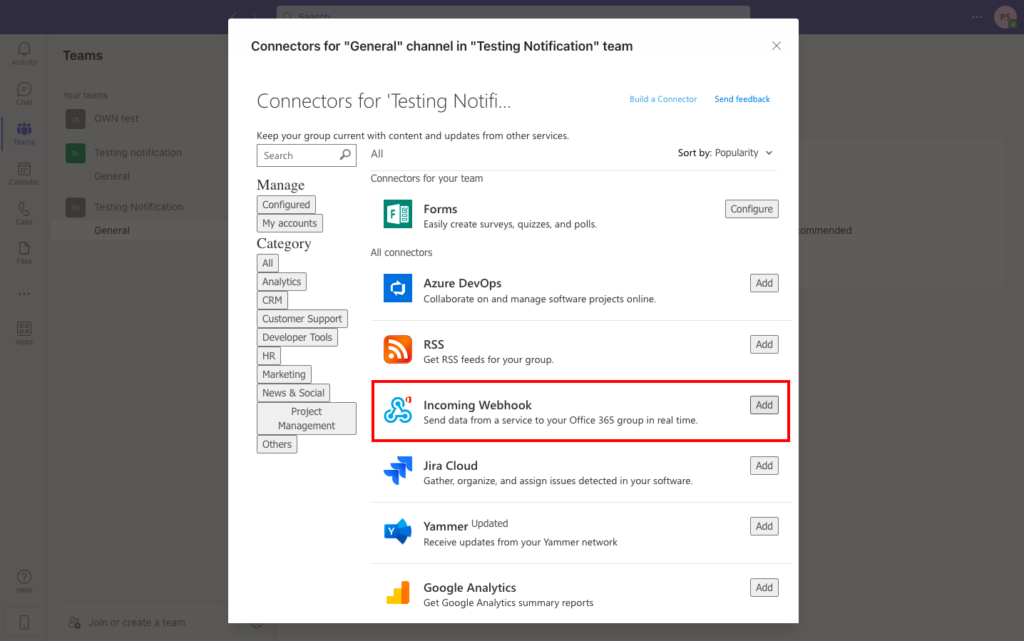
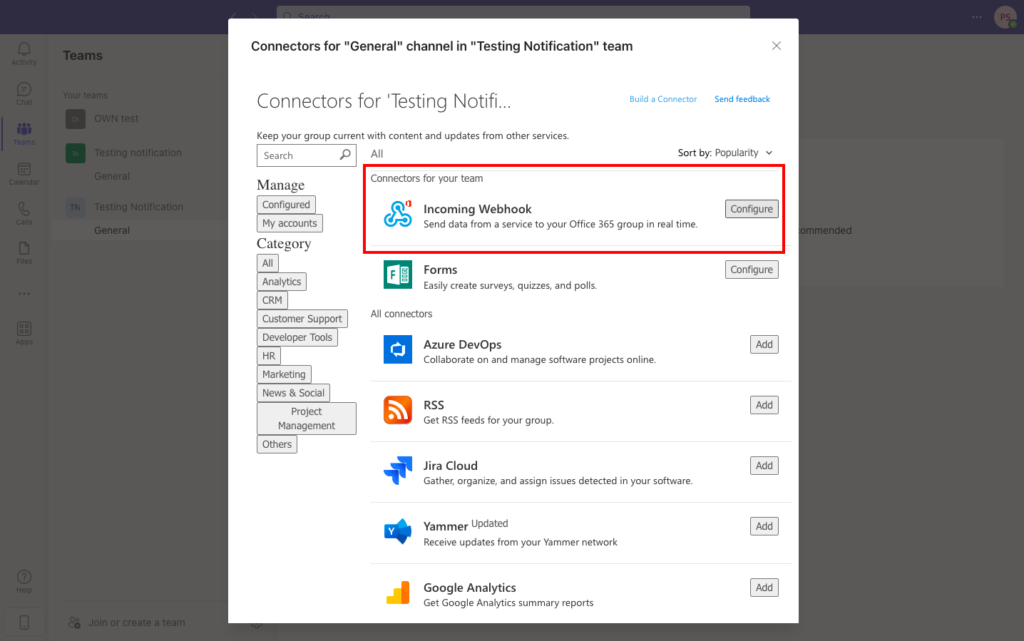
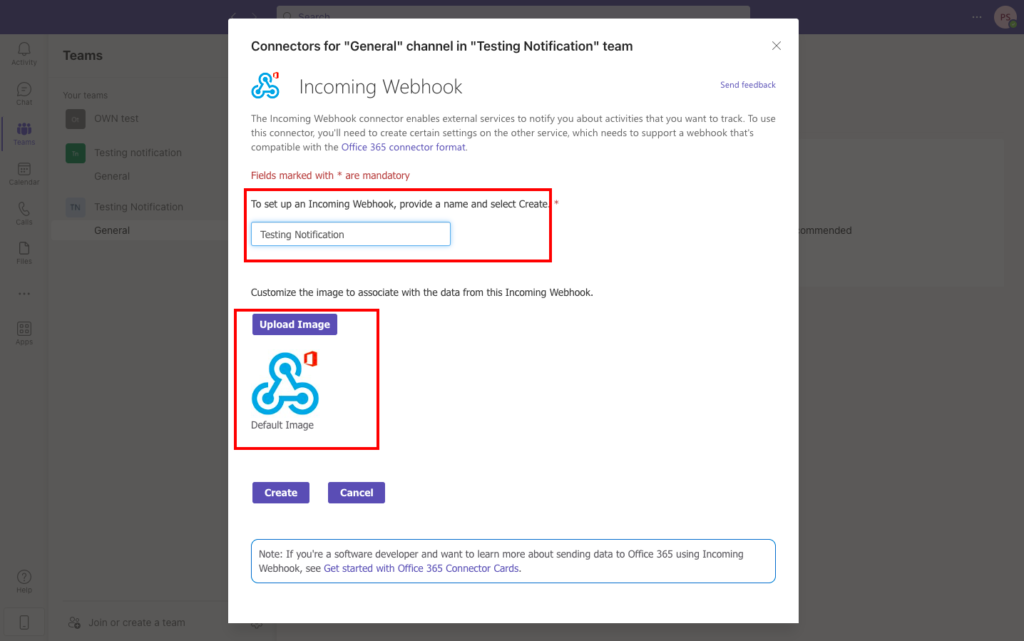
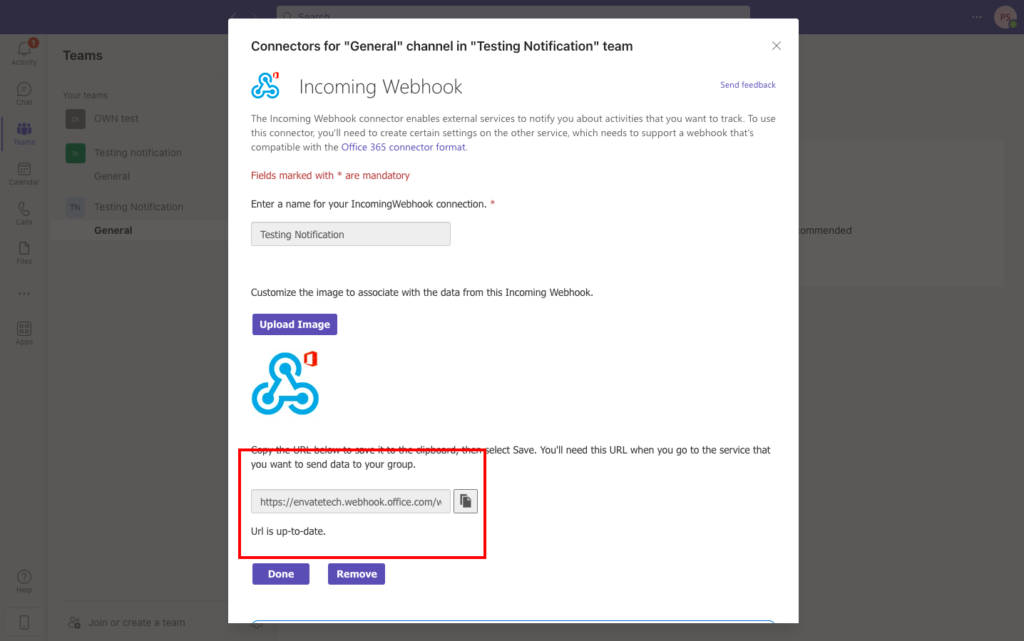
Also Read: Docker Setup Issues: Resolved
Installation (Integration)
To install the Microsoft Teams Notifications Channel for Laravel package in your laravel project, run the following command in your terminal. This command will install Microsoft Teams Notifications Channel for Laravel package via using composer.
composer require laravel-notification-channels/microsoft-teams
Next, if you’re using Laravel without auto-discovery, add the service provider to config/app.php
:
'providers' => [
// ...
NotificationChannels\MicrosoftTeams\MicrosoftTeamsServiceProvider::class,
]
Add Teams channel webhook URL in the environment (.env
) file.
TEAMS_CHANNEL_WEBHOOK_URL = "https://abcde.webhook.office.com/webhookb2/9c708a7f-af2f-4e2d-82fe-2akjhf437yf34gf3c@2f9e0a1e-947c-491d-aed8-1495f1bb899d/IncomingWebhook/a9ec3fb6458f4ksdjhf8344r4fjnqwe3ca767/f005bdaf-b4fe-459d-bb39-0c24f80f62ea"
Configure the webhook URL in your project by adding Microsoft Teams Service. To add Microsoft Teams service, add the following code in your config/services.php
file
...
'microsoft_teams' => [
'webhook_url' => env('TEAMS_CHANNEL_WEBHOOK_URL'),
],
...
Also Read: How To Setup Real-Time Synchronization Using WebSocket with Laravel 11, Pusher, & Angular 18?
Sending Message to Channel (Implementation)
Create a Notification
Run the following command to create notification class inside app/Notifications
php artisan make:notification MSNotification
Create Teams message card
Write the logic to create a message card, its type, and the content that is going to be delivered on the Microsoft Teams channel.
<?php
namespace App\Notifications;
use Illuminate\Bus\Queueable;
use Illuminate\Support\Facades\Auth;
use Illuminate\Notifications\Notification;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Notifications\Messages\MailMessage;
use NotificationChannels\MicrosoftTeams\MicrosoftTeamsChannel;
use NotificationChannels\MicrosoftTeams\MicrosoftTeamsMessage;
class MSNotification extends Notification
{
use Queueable;
private $message;
/**
* Create a new notification instance.
*
* @return void
*/
public function __construct($message)
{
$this->message = $message;
}
/**
* Get the notification's delivery channels.
*
* @param mixed $notifiable
* @return array
*/
public function via($notifiable)
{
return [MicrosoftTeamsChannel::class];
}
/**
* Get the mail representation of the notification.
*
* @param mixed $notifiable
* @return \Illuminate\Notifications\Messages\MailMessage
*/
public function toMicrosoftTeams($notifiable)
{
return MicrosoftTeamsMessage::create()
->to(config('services.microsoft_teams.webhook_url'))
->type('success')
->content($this->message);
}
/**
* Get the array representation of the notification.
*
* @param mixed $notifiable
* @return array
*/
public function toArray($notifiable)
{
return [
//
];
}
}
Also Read: What is N+1 Query Problem? How do you solve it in Laravel?
Send message to the Teams channel
A. Create login route.
<?php
// routes/api.php
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Route;
Route::post('/login', 'Api\ApiController@login');
B. Create controller and method to send message to the Microsoft Teams Channel
<?php
// app/Http/Controllers/Api/ApiController.php
namespace App\Http\Controllers\Api;
use Illuminate\Http\Request;
use F9Web\ApiResponseHelpers;
use App\Http\Controllers\Controller;
use Illuminate\Support\Facades\Auth;
use App\Notifications\MSNotification;
use NotificationChannels\MicrosoftTeams\MicrosoftTeamsChannel;
class ApiController extends Controller
{
use ApiResponseHelpers;
public function login(Request $request)
{
$data = $request->validate([
'email' => 'email|required',
'password' => 'required'
]);
if (!auth()->attempt($data)) {
return response(['error_message' => 'Incorrect Details. Please try again']);
}
$accessToken = auth()->user()->createToken('API Token')->plainTextToken;
$MSmessage = 'Hi '.Auth::user()->fname.'! You are successfully loggedin.';
Notification::route(MicrosoftTeamsChannel::class,null)->notify(new MSNotification($MSmessage));
return $this->setDefaultSuccessResponse([])->respondWithSuccess(['user' => auth()->user(), 'access_token' => $accessToken]);
}
}
Also Read: Setting Up and Configuring Supervisor for Process Management on AWS EC2 Ubuntu
Final Words
Now you know how to send customized messages on the occurrence of different events to the Microsoft Teams Channel from your Laravel project.