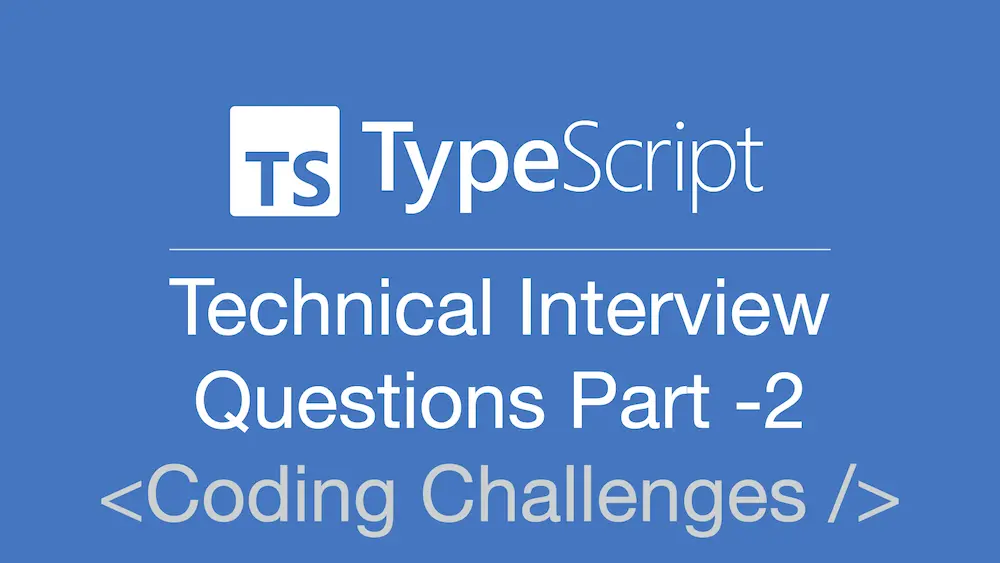
Last Updated On - February 19th, 2024 Published On - Apr 09, 2023
Overview
It’s common for a web developer to go through a coding challenge round in his interview process. In this blog article, I will cover a few typical coding challenges that you may face in any technical interview round when looking for a job. As the popularity of Typescript is growing nowadays I am going to provide you with some popular TypeScript interview questions and their solutions that will help you in cracking the interview.
Coding Challenges
- Create a function that takes in an array of numbers and returns the sum of all the even numbers in the array.
- Implement a function that takes in a number and returns the factorial of that number.
- Implement a function that checks if a given string is a palindrome (i.e. reads the same backward and forward).
- Write a function that takes in a string and returns the number of vowels in the string.
- Create a function that takes in an array of numbers and returns a new array with all the values doubled.
- Write a function that takes in an array of strings and returns the longest string in the array.
- Implement a function that takes in a string and capitalizes the first letter of each word in the string.
- Create a function that takes in an array of numbers and returns the second largest number in the array.
- Write a function that takes in two arrays of numbers and returns a new array with the sum of each pair of corresponding elements.
- Create a function that takes in an array of numbers and returns the largest and smallest numbers in the array.
Also Read: Typescript Technical Interview Questions 2024 – Part 1
Solutions
1. Create a function that takes in an array of numbers and returns the sum of all the even numbers in the array.
function sumOfEvenNumbers(numbers: number[]): number {
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] % 2 === 0) {
sum += numbers[i];
}
}
return sum;
}
// Example usage
const numbers = [1, 5, 12, 6, 9, 8, 21, 28, 17, 4];
const sumOfEvens = sumOfEvenNumbers(numbers); // Returns 58
console.log("Sum of even numbers is: "+sumOfEvens);
Output

2. Implement a function that takes in a number and returns the factorial of that number.
function factorial(num: number): number {
if (num === 0 || num === 1) {
return 1;
}
let result = 1;
for (let i = 2; i <= num; i++) {
result *= i;
}
return result;
}
// Example usage
let num = 5;
let factorialNum = factorial(num); // Returns 120
console.log("The factorial of "+num+" is: "+factorialNum);
num = 11;
factorialNum = factorial(num); // Returns 39916800
console.log("The factorial of "+num+" is: "+factorialNum);
Output

Also Read: Javascript Interview Questions For Frontend Developers 2024 – Part 1
3. Implement a function that checks if a given string is a palindrome (i.e. reads the same backward and forward).
function isPalindrome(str: string): boolean {
str = str.toLowerCase();
for (let i = 0; i < Math.floor(str.length / 2); i++) {
if (str[i] !== str[str.length - 1 - i]) {
return false;
}
}
return true;
}
// Example usage
const str1 = 'racecar';
const str2 = 'Prashant';
const isStr1Palindrome = isPalindrome(str1); // Returns true
const isStr2Palindrome = isPalindrome(str2); // Returns false
console.log(str1+" is a palindrome? "+isStr1Palindrome);
console.log(str2+" is a palindrome? "+isStr2Palindrome);
Output

4. Write a function that takes in a string and returns the number of vowels in the string.
function countVowels(str: string): number {
const vowels = ['a', 'e', 'i', 'o', 'u'];
let count = 0;
for (let i = 0; i < str.length; i++) {
if (vowels.indexOf(str[i].toLowerCase())>=0) {
count++;
}
}
return count;
}
// Example usage
let str = 'Education';
let numVowels = countVowels(str); // Returns 5
console.log({'Given String':str, 'Total number of vowels':numVowels});
str = 'Prashant Singh';
numVowels = countVowels(str); // Returns 3
console.log({'Given String':str, 'Total number of vowels':numVowels});
Output

5. Create a function that takes in an array of numbers and returns a new array with all the values doubled.
function doubleArrayValues(numbers: number[]): number[] {
const doubledNumbers = [];
for (let i = 0; i < numbers.length; i++) {
doubledNumbers.push(numbers[i] * 2);
}
return doubledNumbers;
}
// Example usage
const numbers = [7,13,5,21,64,39];
const doubledNumbers = doubleArrayValues(numbers); // Returns [14, 26, 10, 42, 128, 78]
console.log(doubledNumbers);
Output

Also Read: Laravel Image Resize Project in 10 Minutes
6. Write a function that takes in an array of strings and returns the longest string in the array.
function findLongestString(strings: string[]): string {
let longestString = '';
for (let i = 0; i < strings.length; i++) {
if (strings[i].length > longestString.length) {
longestString = strings[i];
}
}
return longestString;
}
// Example usage
const strings = ['Prashant', 'Sushant', 'Siddhant', 'Ramakant','Vedant'];
const longestString = findLongestString(strings); // Returns 'Prashant'
console.log({'Input Array':strings,'The longest string in the given array is':longestString});
Output
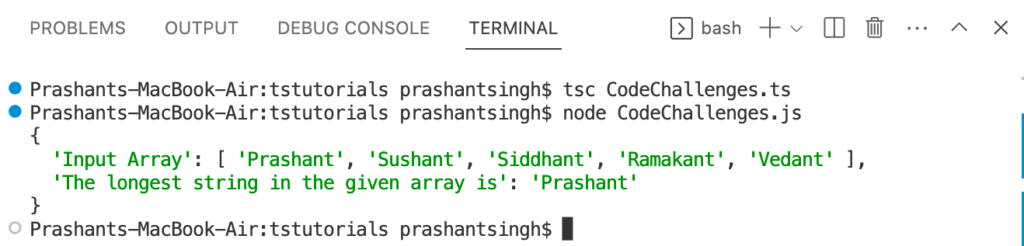
7. Implement a function that takes in a string and capitalizes the first letter of each word in the string.
function capitalizeWords(str: string): string {
const words = str.split(' ');
const capitalizedWords = [];
for (let i = 0; i < words.length; i++) {
capitalizedWords.push(words[i][0].toUpperCase() + words[i].slice(1));
}
return capitalizedWords.join(' ');
}
// Example usage
const str = 'now I can crack the typescript codding challanges';
// Returns 'Now I Can Crack The Typescript Codding Challanges'
const capitalizedStr = capitalizeWords(str);
console.log({'Input String':str,'Converted Capitalize String':capitalizedStr});
Output
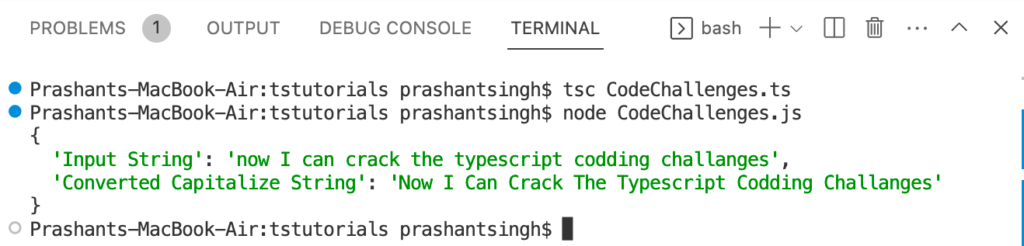
8. Create a function that takes in an array of numbers and returns the second largest number in the array.
function findSecondLargestNumber(numbers: number[]): number {
let largest = numbers[0];
let secondLargest = NaN;
for (let i = 1; i < numbers.length; i++) {
if (numbers[i] > largest) {
secondLargest = largest;
largest = numbers[i];
} else if (numbers[i] > secondLargest && numbers[i] !== largest) {
secondLargest = numbers[i];
}
}
return secondLargest;
}
// Example usage
const numbers = [5, 3, 8, 1, 9, 2, 7, 4, 6];
const secondLargest = findSecondLargestNumber(numbers); // Returns 8
console.log({'Input Array': numbers,'Second largest number in the array is':secondLargest});
Output
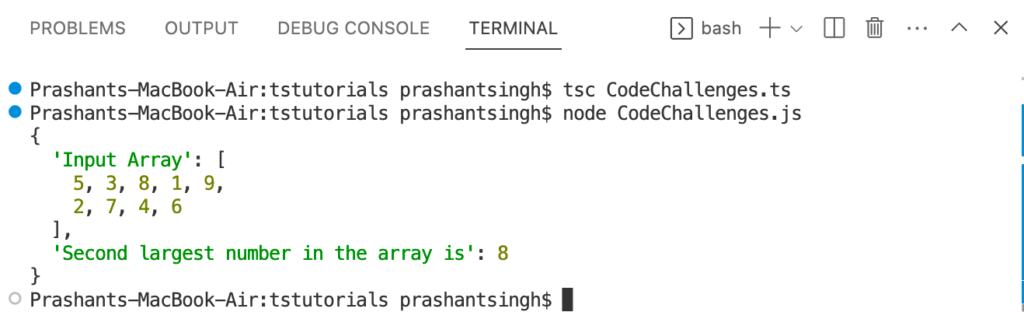
Also Read: Create Short URL Hashing & Tracking Project in 10 Minutes
9. Write a function that takes in two arrays of numbers and returns a new array with the sum of each pair of corresponding elements.
function sumArrays(arr1: number[], arr2: number[]): number[] {
const result = [];
if(arr1.length != arr2.length){
if(arr1.length > arr2.length){
for (let i = 0; i < (arr1.length-arr2.length); i++) {
arr2.push(0); // Making arr2 length equal to arr1 by adding 0 to the arr2
}
}else{
for (let i = 0; i < (arr2.length-arr1.length); i++) {
arr1.push(0); // Making arr1 length equal to arr2 by adding 0 to the arr2
}
}
}
for (let i = 0; i < arr1.length; i++) {
result.push(arr1[i] + arr2[i]);
}
return result;
}
// Example usage
const arr1 = [6, 57, 89, 28, 79];
const arr2 = [35, 46, 12, 44, 84,62];
const sumArray = sumArrays(arr1, arr2); // Returns [41, 103, 101, 72, 163, 62]
console.log({'Input Array 1':arr1,'Input Array 2': arr2,'Sum of each pair of corresponsing values':sumArray});
Output
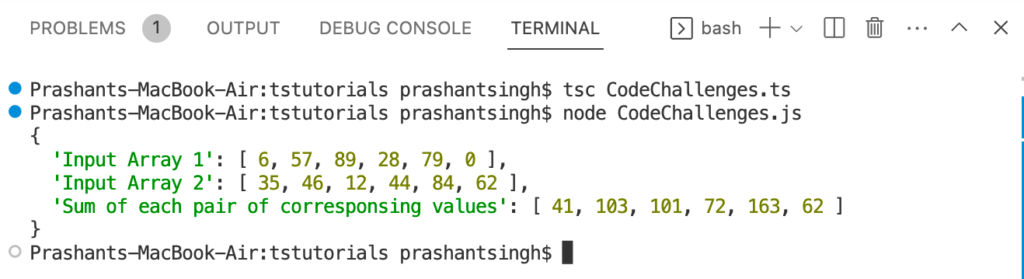
10. Create a function that takes in an array of numbers and returns the largest and smallest numbers in the array.
function findMinMax(numbers: number[]): { min: number, max: number } {
let min = numbers[0];
let max = numbers[0];
for (let i = 1; i < numbers.length; i++) {
if (numbers[i] < min) {
min = numbers[i];
} else if (numbers[i] > max) {
max = numbers[i];
}
}
return { min, max };
}
// Example usage
const numbers = [5, 3, 8, 1, 9, 2, 7, 4, 6];
const { min, max } = findMinMax(numbers); // Returns { min: 1, max: 9 }
console.log({Input:numbers});
console.log({Minimum:min});
console.log({Maximum:max});
Output
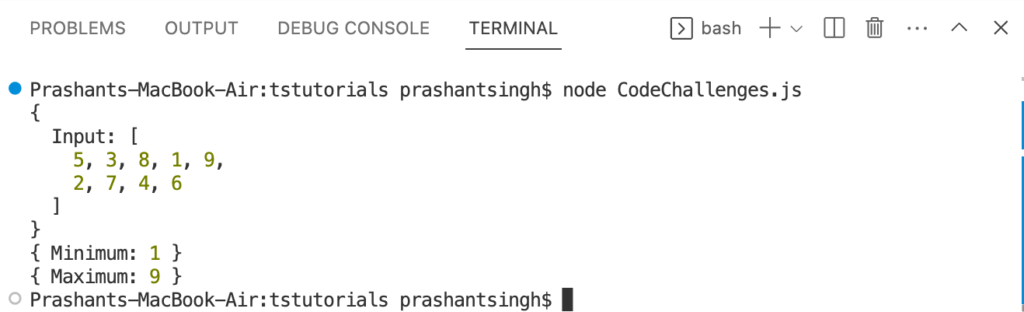
Also Read: Top 10 Python Skills to Make Money in 2023
Conclusion
These are a few coding challenges that you may face in your next technical interview round. It’s crucial to have logical control over the programming to resolve actual issues.