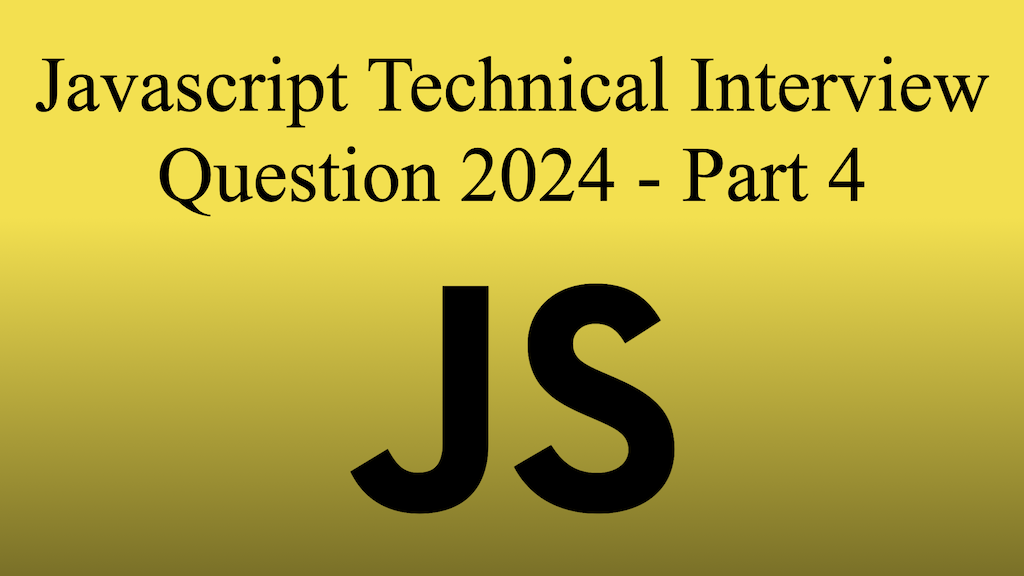
Last Updated On - April 21st, 2024 Published On - Mar 28, 2024
Overview
This is the final episode of JavaScript Interview Questions 2024 series. In this post, I’ll explore some more advanced Javascript Interview Questions. Here are previous episodes of this series Javascript Interview Questions For Frontend Developers 2024 – Part 1 and Javascript Interview Questions 2024 – Part 2, Ace Your Next Interview: Top JavaScript Interview Questions (2024 Update) please check them out first. This series contains some of the latest, trending, and most important questions related to the basics and advanced concepts of javascript, which may help you to understand the core concept of javascript and you’ll be able to crack your next technical interview round.
Q1: What is the DOM?
JavaScript’s DOM: Bringing Your Webpages to Life!
Imagine a play on stage. The actors, props, and background scenery all work together to create the performance. The DOM (Document Object Model) in JavaScript is similar. It’s a way for JavaScript to interact with and manipulate the structure and content of a web page. Here’s how it works:
- The Blueprint (HTML): Think of the play’s script as the blueprint. Just like a play has a script that defines the characters, dialogue, and setting, a web page has HTML that defines its structure (headings, paragraphs, images, etc.).
- The Actors and Props (HTML Elements): The actors and props on stage are like the HTML elements that make up your web page. These elements could be headings, paragraphs, buttons, images, and more.
- The Stage Director (JavaScript): This is where JavaScript comes in! The DOM allows JavaScript to act like the stage director. It can manipulate the HTML elements (actors and props) to change the content, style, and behavior of your web page.
Example
<h1>Welcome!</h1> <button>Click me!</button> <script>
// Get a reference to the button element using its ID
const button = document.getElementById("myButton");
// Add a click event listener to the button
button.addEventListener("click", function() {
alert("You clicked the button!");
});
</script>
In this example, JavaScript uses the DOM to:
- Find the button element with the ID “myButton”.
- Add a click event listener to the button.
- When the button is clicked, it displays an alert message.
Benefits of Using the DOM:
- Dynamic Web Pages: The DOM lets you create interactive and dynamic web pages that can respond to user actions and update content.
- Content Manipulation: You can add, remove, and modify the content of your web page using JavaScript and the DOM.
- Style Changes: You can change the style (color, font size, etc.) of HTML elements using JavaScript and the DOM.
Remember, the DOM is a fundamental concept for making web pages interactive and dynamic using JavaScript. It’s like the stage director, controlling the actors and props on the web page stage!
Also Read: Conquer Docker Setup: Troubleshooting Common Issues on Mac & Linux
Q2: How do you select elements with Vanilla JavaScript?
Selecting Elements Like a Pro: Vanilla JavaScript to the Rescue!
Vanilla JavaScript, the core language without external libraries, offers powerful tools to select elements on your web page. Here are the main methods you can use:
- getElementById:
- Think of this as having a unique ID tag for each element on your stage (web page).
getElementById
is like a spotlight that finds the element with a specific ID you provide. It returns a single element.
<h1 id="main-heading">This is the main heading</h1>
const mainHeading = document.getElementById("main-heading");
console.log(mainHeading); // Outputs the h1 element
2. getElementsByTagName:
- Imagine you want to find all the actors (elements) of a specific type on stage.
getElementsByTagName
acts like a net that catches all the elements with a matching HTML tag name (like “h1”, “p”, “button”). It returns a collection of elements (NodeList) you can loop through.
<p>This is a paragraph.</p>
<p>This is another paragraph.</p>
const paragraphs = document.getElementsByTagName("p");
console.log(paragraphs); // Outputs a NodeList of all paragraphs
3. querySelector:
- This is like having a powerful searchlight that can find elements based on various criteria (ID, class, tag name, etc.).
querySelector
uses CSS selectors (similar to what you use in CSS) to target specific elements. It returns the first matching element.
<div class="special-box">This is a special box</div>
const specialBox = document.querySelector(".special-box");
console.log(specialBox); // Outputs the div element with class "special-box"
4. querySelectorAll:
- Similar to
querySelector
, but it returns a NodeList containing all matching elements, not just the first one. - This is like casting a wide net to find all elements that match your selector.
<button class="action-button">Action 1</button>
<button class="action-button">Action 2</button>
const actionButtons = document.querySelectorAll(".action-button");
console.log(actionButtons); // Outputs a NodeList of all buttons with class "action-button"
Remember:
- Choose the method that best suits your needs.
getElementById
is fast for unique IDs, whilegetElementsByTagName
andquerySelectorAll
are better for finding multiple elements. querySelector
andquerySelectorAll
offer more flexibility with CSS selectors.
By mastering these selection methods, you can control the elements on your web page and create dynamic experiences using Vanilla JavaScript!
Q3: Explain event delegation in JavaScript.
Event Delegation in JavaScript: Handling Events Like a Master!
Ever felt overwhelmed by adding event listeners to a bunch of similar elements in JavaScript? Event delegation is a powerful technique that helps you handle events more efficiently.
Imagine you have a crowded theater with many rows of seats. Instead of assigning a separate usher (event listener) to each seat, event delegation allows you to have a single usher at the entrance (parent element) who checks tickets (event target) and directs attendees (handles events) to their assigned seats (child elements).
Here’s how event delegation works:
- Attaching the Listener: You attach a single event listener to a parent element that contains all the child elements you’re interested in. This is like having the usher stand at the theater entrance.
- Event Bubbling: When an event (like a click) occurs on a child element, it bubbles up the DOM tree. It’s like the attendee going through the entrance first.
- Checking the Target: Inside the event listener function, you use the
event.target
property to identify the specific element that triggered the event. This is like the usher checking the attendee’s ticket. - Handling the Event: Based on the
event.target
, you can perform the desired action for that specific element. This is like the usher directing the attendee to their seat.
Example
<ul id="button-list">
<li><button>Button 1</button></li>
<li><button>Button 2</button></li>
<li><button>Button 3</button></li>
</ul>
<script>
const buttonList = document.getElementById("button-list");
buttonList.addEventListener("click", function(event) {
if (event.target.tagName === "BUTTON") {
console.log("You clicked button:", event.target.textContent);
}
});
</script>
In this example, we have a single event listener on the buttonList
(parent element). When a button is clicked (child element), the event bubbles up, and the event listener checks the event.target
to see if it’s a button. If so, it logs the clicked button’s text content.
Benefits of Event Delegation:
- Improved Performance: Reduces the number of event listeners needed, making your code more performant.
- Dynamic Content: Works well with dynamically added elements, as the event listener is on the parent, which already exists.
- Cleaner Code: Less code duplication compared to adding individual event listeners to each child element.
Remember:
Event delegation is a valuable technique for handling events efficiently in JavaScript, especially when dealing with many similar elements or dynamic content. It helps you write cleaner, more performant code.
Also Read: How to Integrate Google Calendar API Using Laravel 10 to Send Bulk Invites In 2024?
Q4: What is the purpose of the addEventListener method?
In JavaScript, the addEventListener
method is a fundamental tool for creating interactive web pages. It allows you to attach event listeners to elements in the Document Object Model (DOM), enabling your web page to respond to user actions and other events.
Here’s a breakdown of its purpose:
- Event Listeners: These are essentially functions that wait for a specific event (like a click, mouse hover, or key press) to occur on a particular element.
- Attaching Listeners: The
addEventListener
method acts like glue, attaching these event listener functions to DOM elements. You specify the element you want to listen to, the event you’re interested in (like “click”), and the function that should be executed when that event happens.
Example
<button id="myButton">Click me!</button>
<script>
const button = document.getElementById("myButton");
function handleClick() {
alert("You clicked the button!");
}
button.addEventListener("click", handleClick);
</script>
In this example:
- We first get a reference to the button element using its ID.
- Then, we define a function
handleClick
that displays an alert message. - Finally, we use
addEventListener
on the button element. We specify:- The event to listen for:
"click"
(when the button is clicked). - The function to call when the event happens:
handleClick
.
- The event to listen for:
Now, whenever the user clicks the button, the handleClick
function is triggered, displaying the alert message.
Benefits Of Using addEventListener:
- Interactivity: It enables you to create dynamic and interactive web pages that respond to user actions and other browser events.
- Event Handling: It provides a structured way to handle different events happening on your web page.
- Code Reusability: You can define event listener functions separately and reuse them for multiple elements or events.
By effectively using addEventListener
, you can bring your web pages to life and create a more engaging user experience.
Q5: How do you create and remove elements in the DOM?
Let’s break down creating and removing elements in the DOM (Document Object Model) using JavaScript in a clear and beginner-friendly way:
Creating Elements
Imagine you’re a web page architect, and the DOM is your building site. Here’s how you can create new elements:
document.createElement()
: This is like having a blueprint library. You use this method to specify the type of element you want to create (e.g., “p” for paragraph, “div” for a division, etc.). It returns a new, empty element.- Setting Properties (Optional): You can customize the new element using properties. For example, you can set the element’s text content, ID, or class name.
- Appending to the DOM: Once you have your element, you need to place it on your web page. You can use methods like:
appendChild(element)
: This adds the element as the last child of an existing element. Think of it as attaching the new element to the end of a section in your building site.insertBefore(element, referenceElement)
: This inserts the element before another element, specified by thereferenceElement
. It’s like carefully placing the new element between existing ones on your site.
Example
const newParagraph = document.createElement("p");
newParagraph.textContent = "This is a new paragraph!";
const existingElement = document.getElementById("main-content");
existingElement.appendChild(newParagraph);
Removing Elements
Now, imagine you need to renovate your web page and remove some elements. Here’s how you can do that:
parentNode
: This property on an element points to its parent element in the DOM hierarchy. Think of it as following the construction chain to find the element’s parent section.removeChild(element)
: This method removes a specified element from its parent. It’s like demolishing the element from your web page building site.
const elementToRemove = document.getElementById("old-element");
elementToRemove.parentNode.removeChild(elementToRemove);
Additional Considerations
- You can also use the
innerHTML
property to set the entire HTML content of an element, but be cautious as it can overwrite existing content and event listeners. - The
createElement
andappendChild
methods are commonly used together to create and add new elements to your web page. - Remember to choose the appropriate method (
appendChild
orinsertBefore
) based on where you want to place the new element.
By mastering these techniques, you can dynamically modify the structure and content of your web pages using JavaScript, making them more interactive and engaging for users!
Also Read: How to send notification to Microsoft Teams from Laravel 10?
Q6: Explain the concept of event propagation.
In JavaScript, event propagation refers to the mechanism that determines how events travel through the Document Object Model (DOM) when they occur on a web page element. It dictates the order in which elements have the chance to handle the event.
Imagine you have a nested set of boxes, representing the DOM structure. An event, like a click, originates from the innermost box (target element). Event propagation defines the path this click event follows as it bubbles up or trickles down the DOM hierarchy.
There are two main phases of event propagation:
- Capturing Phase (Less Common): (Trickles down) This phase is rarely used but allows you to intercept events before they reach the target element. Event listeners set with the
useCapture
parameter set totrue
are triggered during this phase. - Bubbling Phase (Default): (Bubbles up) This is the more common phase. When an event occurs on an element, it first triggers any event listeners on that element (the target). Then, the event bubbles up through its ancestors (parent elements, grandparents, and so on) until it reaches the document object (the outermost element). Event listeners on these ancestor elements are triggered in the order they appear in the DOM tree.
Here’s a breakdown of the key points:
- Target Element: The element where the event originates (the innermost box in our analogy).
- Event Bubbling: The default behavior where events travel up the DOM tree, triggering listeners on ancestor elements.
- Event Capturing: The less common phase where events can be listened to before reaching the target element, trickling down from the document object.
Why is Event Propagation Important?
Understanding event propagation is crucial for several reasons:
- Handling Events Efficiently: You can leverage bubbling to avoid adding event listeners to every element. By placing a listener on a parent element, you can handle events for all its children.
- Complex Interactions: Event propagation allows you to create intricate interactions between elements. For example, clicking a button inside a menu might also trigger an event on the menu itself.
- Event Stopping: You can control propagation using methods like
stopPropagation
to prevent an event from bubbling up further after it’s been handled.
By mastering event propagation, you can write cleaner, more efficient, and interactive JavaScript code for your web applications.
Q7: How can you prevent the default behavior of an event?
In JavaScript, preventing the default behavior of an event gives you more control over how a web page reacts to user interactions. By default, certain events like clicks or form submissions might trigger built-in behaviors (like following a link or submitting a form). You can use JavaScript to stop these defaults and create custom actions instead.
Here’s how you can prevent the default behavior of an event:
- Event Object: When an event listener function is triggered, it receives an event object as an argument. This object contains information about the event, including methods to control its behavior.
preventDefault()
Method: This method, available on the event object, is the key to stopping the default behavior. Callevent.preventDefault()
inside your event listener function to prevent the browser’s default action for that event.
Example
<a href="#" id="myLink">Click me (default prevented)</a>
<script>
const myLink = document.getElementById("myLink");
myLink.addEventListener("click", function(event) {
// Prevent the default behavior (following the link)
event.preventDefault();
// Your custom action here (e.g., display an alert)
alert("You clicked the link, but we prevented the default behavior!");
});
</script>
In this example:
- We have a link with an ID
myLink
. - The click event listener prevents the default behavior (
event.preventDefault()
) when the link is clicked. - Instead, a custom alert message is displayed.
Important Considerations
- Preventing default behavior is useful for creating custom interactions and handling form submissions without reloading the page.
- Use it judiciously, as some default behaviors might be expected by users (e.g., preventing a link from navigating away might be confusing).
Additional Methods
- You might also encounter the
returnValue
property (older approach) for preventing defaults, butpreventDefault
is the more modern and recommended way. - Some events, like
focus
orblur
, don’t have a default behavior to prevent.
By understanding how to prevent default event behavior, you can create more interactive and engaging web experiences using JavaScript.
Also Read: PNPM vs NPM: Why should we use PNPM over NPM?
Q8: What is the purpose of the data- attribute in HTML?
The data-*
attribute in HTML is a versatile tool that allows you to store custom data directly on HTML elements without affecting the element’s functionality or appearance. It provides a way to associate private information with an element for use by scripts on the same page, or potentially by server-side code.
Here’s a breakdown of its key characteristics:
Structure
- The
data-*
attribute always starts withdata-
followed by a hyphen (-). - The rest of the attribute name is typically a descriptive identifier related to the data being stored. You can use camelCase or kebab-case for the custom name (e.g.,
data-productId
,data-user-preference
).
Purpose
- Stores private data that isn’t directly relevant to the element’s content or visual presentation. This can include things like:
- Unique identifiers for elements (e.g.,
data-product-id="123"
) - User preferences (e.g.,
data-theme="dark"
) - Flags or states (e.g.,
data-is-active="true"
) - Information for client-side scripts (e.g., data for charts or maps)
- Unique identifiers for elements (e.g.,
Benefits
- Semantic Neutrality: Doesn’t affect the element’s meaning or presentation in the browser. Screen readers and crawlers typically ignore
data-*
attributes. - Flexibility: You can store a wide variety of data types as strings using
data-*
attributes. - JavaScript Access: JavaScript can easily access and manipulate the data using the
dataset
property of the element.
Example
<img src="image.jpg" alt="Product Image" data-product-id="456">
In this example, the data-product-id
attribute stores the product ID (456) associated with the image. A JavaScript function could later retrieve this ID using the dataset
property.
const imageElement = document.querySelector("img");
const productId = imageElement.dataset.productId; // Accessing data using dataset
console.log(productId); // Outputs: "456"
Things to Consider
- While
data-*
attributes are convenient for private data, avoid using them for critical information that needs stronger data validation or persistence. - For more complex data structures, consider using alternative solutions like JSON or dedicated JavaScript libraries.
By effectively using data-*
attributes, you can enhance the functionality of your web pages and create a more dynamic user experience without cluttering your HTML with presentational details.
Q9: Describe the difference between innerHTML and textContent.
Both innerHTML
and textContent
are properties in JavaScript used to manipulate the content of HTML elements, but they deal with that content in fundamentally different ways. Here’s a breakdown to clarify their distinctions:
innerHTML
- Represents: The entire HTML code contained within an element, including all child elements and their text content, tags, and attributes.
- Behavior:
- Getting: Returns a string of the complete HTML content within the element.
- Setting: Replaces the entire inner content of the element with the provided HTML string. This can be dangerous if not handled carefully, as it can overwrite existing event listeners and lead to XSS (Cross-Site Scripting) vulnerabilities if you’re setting user-generated content.
textContent
- Represents: The combined text content of an element and all its child elements, but it excludes any HTML tags or attributes.
- Behavior:
- Getting: Returns a string of the combined text content, including whitespace (spaces, newlines) by default.
- Setting: Replaces the entire text content of the element and its children with the provided string. This is generally safer for setting content as it doesn’t allow for unintended script execution.
Key Differences
Feature | innerHTML | textContent |
---|---|---|
Content Type | Entire HTML code within the element | Combined text content, excluding tags and attributes |
Tags and Attributes | Included | Excluded |
Whitespace (default) | Preserved | Preserved |
Setting Safety | Can be risky (potential XSS vulnerabilities) | Generally safer |
When to Use Which
- Use
innerHTML
cautiously when you specifically need to modify the element’s complete HTML structure, including tags and attributes. Be mindful of XSS risks when setting content from external sources. - Use
textContent
in most cases for setting or retrieving the text content of an element. It’s a safer option for updating the content without introducing unintended elements or scripting issues.
Example
<div id="myElement">
This is some <b>bold</b> text with a <span id="special">special span</span>.
</div>
- Using
innerHTML
onmyElement
:- Getting: Would return the entire HTML content as a string, including the
<b>
tag and<span>
element. - Setting: If you set
myElement.innerHTML = "New Content"
, it would replace the entire content of the element with just the text “New Content”, removing the bold formatting and the span element.
- Getting: Would return the entire HTML content as a string, including the
- Using
textContent
onmyElement
:- Getting: Would return the combined text content, which is “This is some bold text with a special span.” (including spaces).
- Setting: If you set
myElement.textContent = "Updated Text"
, it would replace the entire text content within the element, but the bold formatting and span element would still be there.
Remember
Choose the property that best suits your needs based on whether you want to work with the complete HTML structure (including tags) or just the text content. Be cautious when using innerHTML
for setting content, especially from untrusted sources.
Q10: How do you handle asynchronous code in JavaScript?
Ah, asynchronous code! This is a fundamental concept in JavaScript for building dynamic and responsive web applications. Here’s a breakdown to guide you through handling asynchronous operations effectively:
What is Asynchronous Code?
JavaScript, by default, executes code synchronously, meaning one line after the other. But web applications often involve waiting for external factors like:
- Fetching data from a server (using APIs or AJAX)
- User interactions (like button clicks or form submissions)
- Timers (like
setTimeout
orsetInterval
) - File operations (in Node.js environments)
These are asynchronous operations because JavaScript can’t continue execution and wait for them to finish before moving on.
How to Handle Asynchronous Code
There are two main approaches to dealing with asynchronous code in JavaScript:
Callbacks:
- The traditional approach. You pass a function (the callback) as an argument to the asynchronous function.
- When the asynchronous operation finishes, the callback function is executed with the result (or error) as an argument.
function fetchData(callback) {
// Simulate asynchronous operation (e.g., API call)
setTimeout(() => {
const data = { name: "John", age: 30 };
callback(data); // Call the callback function with the data
}, 1000);
}
fetchData(function(data) {
console.log("Data received:", data);
});
Promises:
- A more modern approach that provides better readability and error handling.
- An asynchronous operation returns a Promise object, which represents the eventual completion (or failure) of the operation.
- You can use the
then
andcatch
methods on the Promise to handle the successful result or any errors that might occur.
function fetchData() {
return new Promise((resolve, reject) => {
// Simulate asynchronous operation (e.g., API call)
setTimeout(() => {
const data = { name: "John", age: 30 };
resolve(data); // Resolve the promise with the data
}, 1000);
});
}
fetchData()
.then(data => console.log("Data received:", data))
.catch(error => console.error("Error:", error));
Async/Await (ES6+):
- Builds on top of Promises and provides a cleaner syntax for writing asynchronous code.
- You can use the
async
keyword before a function to declare it as asynchronous. - Inside an async function, you can use the
await
keyword before a Promise to pause execution until the Promise resolves (or rejects).
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log("Data received:", data);
} catch (error) {
console.error("Error:", error);
}
}
fetchData();
Choosing the Right Approach
- Callbacks are a good starting point, but they can lead to nested callback hell for complex asynchronous operations.
- Promises offer better code organization and error handling.
- Async/await provides the most concise and readable way to write asynchronous code (available in ES6 and later environments).
Additional Tips
- Always handle errors in your asynchronous code using
try...catch
blocks or promise rejections. - Consider using libraries like Axios for easier HTTP requests and handling asynchronous operations.
By effectively handling asynchronous code, you can build responsive and user-friendly web applications that don’t lock up the browser while waiting for external data or user interactions.