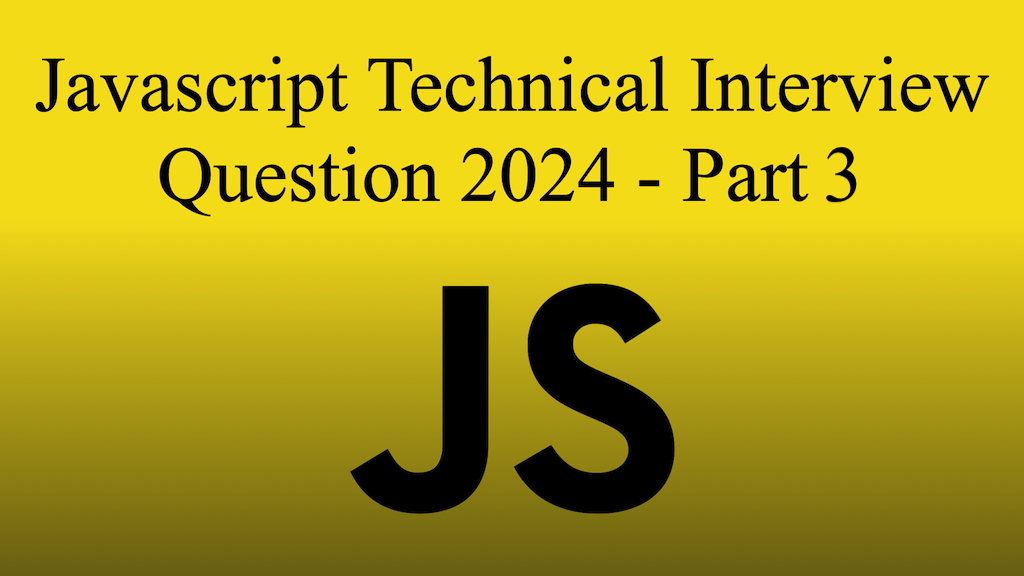
Last Updated On - March 15th, 2024 Published On - Mar 09, 2024
Overview
In this post, I’ll explore some advanced Javascript Interview Questions which is a part of the Javascript Interview Questions 2024 series. If you didn’t go through the previous parts i.e. Javascript Interview Questions For Frontend Developers 2024 – Part 1 and Javascript Interview Questions 2024 – Part 2 please check them out first. This series contains some of the latest, trending, and most important questions related to the basics and advanced concepts of javascript, which may help you to understand the core concept of javascript and you’ll be able to crack your next technical interview round.
Q1: What is prototypal inheritance?
Imagine you’re building a bunch of cool cars. You wouldn’t build each car from scratch, right? Instead, you’d probably start with a blueprint for a basic car, then customize it for different models.
That’s kind of what prototypal inheritance does in JavaScript. Here’s how it works:
- The Blueprint (Prototype): Every object in JavaScript has a hidden blueprint called a prototype. This blueprint holds properties and methods (like functions) that the object can inherit.
- Inheriting the Cool Stuff: When you create a new object, it inherits properties and methods from its prototype. So, it gets all the cool features from the blueprint without you having to write them again!
- Looking Up the Family Tree: If the new object tries to use a property or method it doesn’t have, JavaScript searches the prototype’s “family tree” to find it. It keeps looking up the chain until it finds the property or reaches the end of the line.
Think of it like this:
// Our Blueprint (Car Prototype)
const car = {
wheels: 4,
makeNoise: function() {
console.log("Beep Beep!");
}
};
// Creating a New Car (SportsCar inherits from car)
const sportsCar = Object.create(car);
sportsCar.color = "Red";
console.log(sportsCar.wheels); // Inherited from blueprint (4)
sportsCar.makeNoise(); // Inherited function (Beep Beep!)
Here, sportsCar
inherits the wheels
property and makeNoise
function from the car
blueprint.
This way, you can create all sorts of new objects (like different car models) with the same basic features (wheels, making noise) and add their own unique properties (color). Pretty neat, right?
Remember, prototypal inheritance is a powerful tool in JavaScript for code reusability and building object relationships.
Q2: How do you create an object in JavaScript?
JavaScript Object Creation: The Easiest Way to Make Your Own Stuff!
Imagine you have a toolbox filled with different tools. In JavaScript, an object is like a special box where you can store your own things, just like the tools in your toolbox. These things can be labels (like “name”) and values (like “Alice”).
Here’s how to create your own object in JavaScript, just like packing your toolbox:
- Curly Braces ({ }): These are like the walls of your box. Put everything you want inside these curly braces.
- Labels and Values (name:value): Separate each item in your box with a comma (,). Each item has two parts: a label (like a name tag) and a value (the actual tool). They’re separated by a colon (:).
For example, let’s create an object to describe your favorite pet:
const myPet = {
name: "Luna", // Label (name) and value ("Luna")
species: "Cat", // Another label (species) and value ("Cat")
age: 2 // One more label (age) and value (2)
};
Here, myPet
is your object, and it has three items inside:
name: "Luna"
tells everyone your pet’s name is Luna.species: "Cat"
tells everyone your pet is a cat.age: 2
tells everyone your pet is 2 years old.
That’s it! You’ve created your own object in JavaScript. Now you can use this object to store information about anything you like, from your favorite toy to your superhero costume!
Remember, objects are like customizable boxes to hold all sorts of cool stuff in your JavaScript programs.
Also Read: Typescript Technical Interview Questions 2024 – Part 1
Q3: What is the purpose of the prototype property in JavaScript?
JavaScript Prototypes Explained: Sharing Superpowers Like a Superhero!
Imagine you’re in a superhero academy, learning amazing skills. The prototype
property in JavaScript is like the master trainer who holds all the secret knowledge (methods and properties) that superheroes can inherit.
Here’s how it works:
- The Master Trainer (Prototype): Every function and object in JavaScript has a hidden
prototype
property. This property acts like a master trainer, containing all the cool superhero moves (methods) and characteristics (properties) that can be passed on. - Learning from the Best (Inheritance): When you create a new object using a constructor function (like creating a new superhero), that object inherits all the powers (methods and properties) from the constructor’s prototype. It’s like getting trained by the master!
- Sharing the Knowledge (Code Reusability): This inheritance sayesinde (say “saanen-dee-yen”), which is Turkish for “thanks to this,” you don’t have to define the same superpowers (methods and properties) for every new object. It saves you time and keeps your code clean.
Here’s an example to show you how it works:
// Master Trainer (Animal Prototype)
function Animal(name) {
this.name = name;
this.makeNoise = function() {
console.log("Generic animal noise!");
}
}
// Creating a New Superhero (Dog inherits from Animal)
const dog = new Animal("Buddy");
console.log(dog.name); // Inherited property ("Buddy")
dog.makeNoise(); // Inherited method (Generic animal noise!)
In this example, the Animal
function acts as the prototype, holding the name
property and the makeNoise
method. When we create a new dog
object, it inherits these powers from the Animal
prototype.
Remember, the prototype
property is a key concept in JavaScript for code reusability and building object relationships. It’s like having a master trainer to share all the coolest superhero skills!
Q4: Explain The Difference Between Object.create
And The Constructor Pattern.
Object.create
vs Constructor Pattern: JavaScript Object Creation Showdown!
Both Object.create
and the constructor pattern are ways to create objects in JavaScript, but they work in slightly different ways. Here’s a kid-friendly explanation:
Imagine you’re building with Legos.
- The Constructor Pattern: Think of the constructor pattern as a big Lego set with instructions. It comes with all the pieces (properties) and a guide (methods) to build a specific kind of object (like a spaceship). You follow the instructions to put the pieces together and create a new spaceship object.
- Object.create: This is like having a box of random Legos. You can use
Object.create
to build a new object by taking Legos (properties) from another existing object (like a spaceship you already built). You can even add new Legos (your own properties) on top.
Here’s a table to show the key differences:
Feature | Constructor Pattern | Object.create |
---|---|---|
Inherits from | Constructor’s prototype | Specified object |
Code execution | Runs constructor code | No code execution |
Example | const car = new Car() | const newCar = Object.create(car) |
Here’s when you might use each one:
- Constructor Pattern: Use this when you want to create many objects of the same type with some pre-defined properties and behaviors (like building a bunch of spaceships).
- Object.create: Use this when you need more flexibility and want to create an object that inherits from a specific existing object, but also want to add your own properties (like making a custom spaceship with extra wings).
Remember, both methods are useful tools for creating objects in JavaScript. Choose the one that best fits your needs for code reusability and customization!
Also Read: Coding Challenge: Typescript Interview Questions 2024 – Part 2
Q5: How do you add a property to an object in JavaScript?
JavaScript Object Power-Up: Adding Cool Stuff with Properties!
Imagine you have a toolbox, but it’s missing some important tools. In JavaScript, objects are like these toolboxes, and properties are the tools you put inside. You can add new properties to an object anytime to give it more functionality!
There are two main ways to add properties to an object:
- Dot Notation: Think of this as using a label maker to name your tools. You use a dot (.) followed by the property name (like “hammer”) and then an equal sign (=) to assign a value (the actual hammer) to the property.
Here’s an example:
const toolbox = {}; // Empty toolbox
toolbox.hammer = ""; // Adding a hammer property with dot notation
console.log(toolbox); // Now toolbox has a hammer!
- Bracket Notation: This is like using sticky notes to label your tools. You use square brackets ([ ]) around the property name (like [“screwdriver”]) and then an equal sign (=) to assign a value (the actual screwdriver). This is useful when the property name has spaces or special characters.
Here’s an example:
const toolbox = {}; // Empty toolbox
toolbox["screw driver"] = "🪛"; // Adding a screwdriver property with bracket notation
console.log(toolbox); // Now toolbox has a screwdriver!
Remember, you can add as many properties as you want to your objects using dot notation or bracket notation. This lets you customize your objects and make them more powerful!
Q6: What is the hasOwnProperty
method used for?
JavaScript’s hasOwnProperty Detective: Who Owns This Property?
Imagine you’re working on a big project with a bunch of tools, and some belong to you, while others are borrowed from your friends. The hasOwnProperty
method in JavaScript acts like a detective, helping you figure out which properties truly belong to your object.
Here’s how it works:
- Checking Ownership: JavaScript objects can inherit properties from other objects (like borrowing tools).
hasOwnProperty
checks if a specific property exists directly on the object itself, not just if it’s inherited from somewhere else. - True or False: This method returns a simple
true
orfalse
answer. If the property truly belongs to the object (it’s not inherited),hasOwnProperty
returnstrue
. If the property is inherited or doesn’t exist at all, it returnsfalse
. - Why it Matters: Knowing if a property is truly owned by the object is important in various situations. For example, you might want to loop through only the object’s own properties, not inherited ones.
Here’s an example to show you how it works:
const car = {
wheels: 4,
makeNoise: function() {
console.log("Beep Beep!");
}
};
const sportsCar = Object.create(car);
sportsCar.color = "Red";
console.log(sportsCar.hasOwnProperty("wheels")); // false (inherited)
console.log(sportsCar.hasOwnProperty("color")); // true (own property)
In this example, sportsCar
inherits the wheels
property from the car
object. So, hasOwnProperty
returns false
for wheels
. However, sportsCar
has its own color
property, so hasOwnProperty
returns true
for color
.
Remember, the hasOwnProperty
method is a handy tool for checking true ownership of properties in JavaScript objects. It helps you be a property ownership detective in your code!
Also Read: Learn MongoDB in 14 Steps: A 10 Minutes Guide
Q7: How can you prevent modification of object properties in JavaScript?
JavaScript Object Lockbox: Keeping Your Data Safe!
Imagine you have a treasure chest filled with valuable stuff, but you want to make sure nobody can mess with it. In JavaScript, objects are like treasure chests, and properties are the valuables inside. There are three ways to act as a guard and prevent modifications:
- Object.preventExtensions: Think of this as putting a heavy lock on the chest. It prevents anyone from adding new properties altogether. Existing properties can still be changed, but no new ones can sneak in.
- Object.seal: This is like putting a special lock that allows looking at the treasure (accessing properties) but not taking anything out (changing property values) or adding anything new (adding properties).
- Object.freeze: This is the ultimate lock! It completely freezes the chest. Nobody can change the values of existing properties, add new ones, or even delete them. The treasure is safe and sound!
Here’s a table to show the key differences:
Feature | Object.preventExtensions | Object.seal | Object.freeze |
---|---|---|---|
Add new properties | No | No | No |
Change existing values | Yes | Yes | No |
Delete properties | Yes | No | No |
Example | Object.preventExtensions(object) | Object.seal(object) | Object.freeze(object) |
Remember:
- Use
Object.preventExtensions
when you only want to restrict adding new properties. - Use
Object.seal
when you want to prevent adding new properties and deleting existing ones, but allow modifying values. - Use
Object.freeze
for complete protection – no modifications allowed!
These methods are helpful for situations where you want to ensure data integrity and prevent accidental changes to your objects.
Q8: Describe the use of the new keyword.
JavaScript’s new Keyword: The Magic Behind Creating Objects
Imagine you have a special blueprint for building amazing robots. The new
keyword in JavaScript acts like a magic tool that brings this blueprint to life, creating a brand new robot object based on your instructions.
Here’s how it works:
- The Blueprint (Constructor Function): In JavaScript, a constructor function is like your robot blueprint. It defines the properties (like height, weight) and methods (like walk, talk) that all your robots will have.
- Using the new Keyword: When you call a function with the
new
keyword in front of it, JavaScript knows you want to create a new object based on that function’s blueprint. It’s like using the magic tool to activate the blueprint! - The New Robot Object: Behind the scenes, JavaScript does several things:
- Creates a brand new, empty object.
- Sets this object’s internal prototype to the constructor function’s prototype (think of it as inheriting traits).
- Executes the code inside the constructor function, allowing you to define properties and methods specifically for this new robot object.
- Finally, returns the newly created robot object for you to use!
Here’s an example to show you what we mean:
function Robot(name, height) {
this.name = name;
this.height = height;
this.greet = function() {
console.log("Beep boop! My name is " + this.name);
};
}
const robot1 = new Robot("RX-78", 18.5); // Using new keyword to create a robot object
console.log(robot1.name); // "RX-78" (property from constructor)
robot1.greet(); // "Beep boop! My name is RX-78" (method from constructor)
In this example, the Robot
function is the blueprint. When we use new Robot("RX-78", 18.5)
, the new
keyword creates a new robot object named robot1
with the specified name and height. It also inherits the greet
method from the blueprint.
Remember, the new
keyword is essential for creating objects based on constructor functions in JavaScript. It’s the magic tool that brings your object blueprints to life!
Also Read: PNPM vs NPM: Why should we use PNPM over NPM?
Q9: Explain the concept of Object Destructuring in JavaScript.
JavaScript Object Destructuring: Unpacking Your Toolbox Like a Pro!
Imagine you have a toolbox filled with all sorts of tools, but you only need a hammer and screwdriver for your project. Object Destructuring in JavaScript is like a special technique to quickly grab the exact tools (properties) you need from a toolbox (object) without taking out everything.
Here’s how it works:
- Matching Names: Think of labeling your tools clearly. In Object Destructuring, you create variables with names that match the property names you want to extract from the object. It’s like having labels ready for the tools you need.
- Destructuring Assignment: This is the magic trick! You use curly braces ({ }) and assign the object properties to your variables directly. It’s like reaching into the toolbox and grabbing only the tools you labeled.
Here’s an example to show you what we mean:
const toolbox = {
hammer: "",
screwdriver: "🪛",
wrench: "",
nails: ""
};
// Traditional way (getting everything):
const allTools = toolbox;
// Destructuring to get only hammer and screwdriver:
const { hammer, screwdriver } = toolbox;
console.log(hammer); // ""
console.log(screwdriver); // "🪛"
In this example, we use destructuring to directly assign the hammer
and screwdriver
properties to separate variables (hammer
and screwdriver
). We don’t need to grab everything from the toolbox (object) at once.
Benefits of Destructuring:
- Cleaner Code: Destructuring makes your code more readable and avoids repetitive property access using dot notation.
- Easier Assignment: You can directly assign properties to variables with matching names, saving time.
- Default Values: You can also set default values for properties that might not exist in the object.
Remember, Object Destructuring is a powerful tool for unpacking objects and grabbing only the specific properties you need in JavaScript. It makes your code cleaner and more efficient!
Q10: What is the difference between null and undefined?
null vs undefined in JavaScript: Don’t Let Them Fool You!
Both null
and undefined
are special values in JavaScript, but they have distinct meanings. Here’s a breakdown for beginners:
- null: Think of
null
as an empty box. It’s a deliberate assignment that indicates a variable doesn’t hold any object or value. It’s like saying, “This box is intentionally empty.” - undefined: Imagine
undefined
as a vacant parking spot. It means a variable has been declared but hasn’t been assigned a value yet. It’s like saying, “This parking spot is reserved, but there’s no car here yet.”
Here’s a table to summarize the key differences:
Feature | null | undefined |
---|---|---|
Assignment | Deliberate assignment (variable = null) | Not assigned a value yet |
Type | Object | undefined |
Equality (== check) | null == undefined evaluates to false | null == undefined evaluates to false |
Strict equality (=== check) | null === undefined evaluates to false | null === undefined evaluates to false |
Here are some additional points to remember:
- You can explicitly assign
null
to a variable:let emptyBox = null;
undefined
is automatically assigned by JavaScript when you declare a variable without a value:let name;
(here,name
is undefined).- Although sometimes they might be treated similarly in certain situations,
null
andundefined
have distinct meanings in JavaScript.
Tip: Using a linter or code formatter can help you avoid accidentally leaving variables undefined!
Summary
In this blog post, we explored a variety of advanced JavaScript interview questions that you might encounter during your next coding challenge. We discussed core JavaScript concepts such as prototypal inheritance, object creation, the constructor pattern, Object.create
, and the hasOwnProperty
method. By understanding these concepts, you’ll be well-equipped to tackle even the most challenging JavaScript interview questions.
To learn more about my web development skills and experience, visit my portfolio: Prashant Web Developer.